Submit buttons
By default, each "step" within your action that requests user input renders a single submit button allowing the person running the action to move on when they've filled out the form.
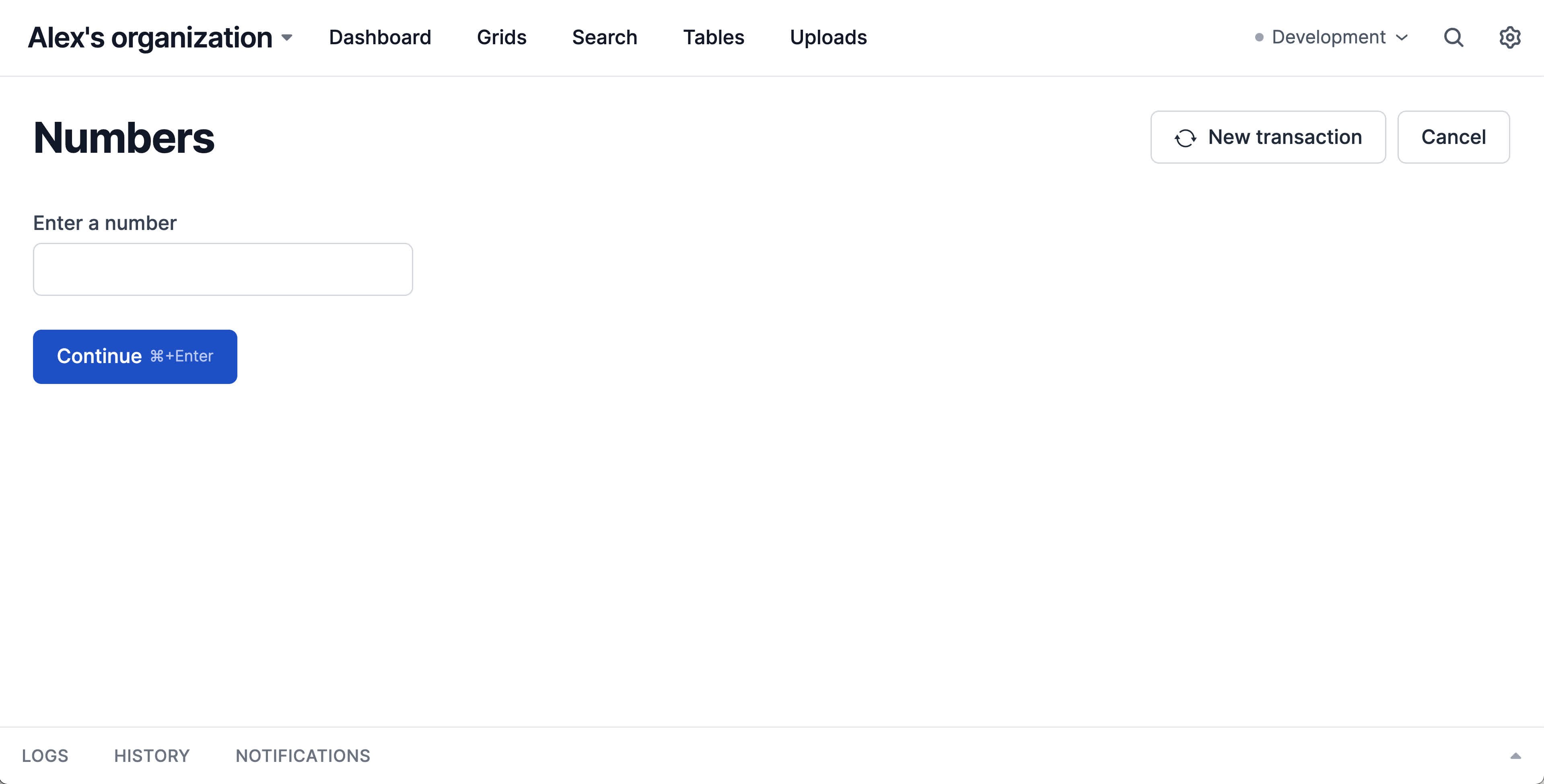
You may want to customize this button, or provide multiple paths forward, allowing for branching logic so that one action can do more than one thing and the person running the action can flexibly change behavior as needed.
To accomplish this, chain any I/O method or group with withChoices()
, and pass an array of options defining the available paths forward. Each provided option will render a button in the action UI, and change the I/O method or group return value to contain a choice
identifying the chosen button in addition to the usual return value(s) from the I/O methods.
Perform any conditional logic or branching based off this choice
value as you would any variable.
To accomplish this, chain any I/O method or group with withChoices()
, and pass an array of options defining the available paths forward. Each provided option will render a button in the action UI, and change the I/O method or group return value to contain a choice
identifying the chosen button in addition to the usual return value(s) from the I/O methods.
Perform any conditional logic or branching based off this choice
value as you would any variable.
To accomplish this, chain any I/O method or group with with_choices()
, and pass an array of options defining the available paths forward. Each provided option will render a button in the action UI, and change the I/O method or group return value to contain a choice
identifying the chosen button in addition to the usual return value(s) from the I/O methods.
Perform any conditional logic or branching based off this choice
value as you would any variable.
- TypeScript
- JavaScript
- Python
let { choice, returnValue } = await io.input
.number("Enter a number")
.withChoices([
"Round up",
{ label: "Make it negative", value: "negate", theme: "secondary" },
{ label: "Divide by zero", value: "divide-by-zero", theme: "danger" },
]);
if (choice === "Round up") {
returnValue = Math.ceil(returnValue);
}
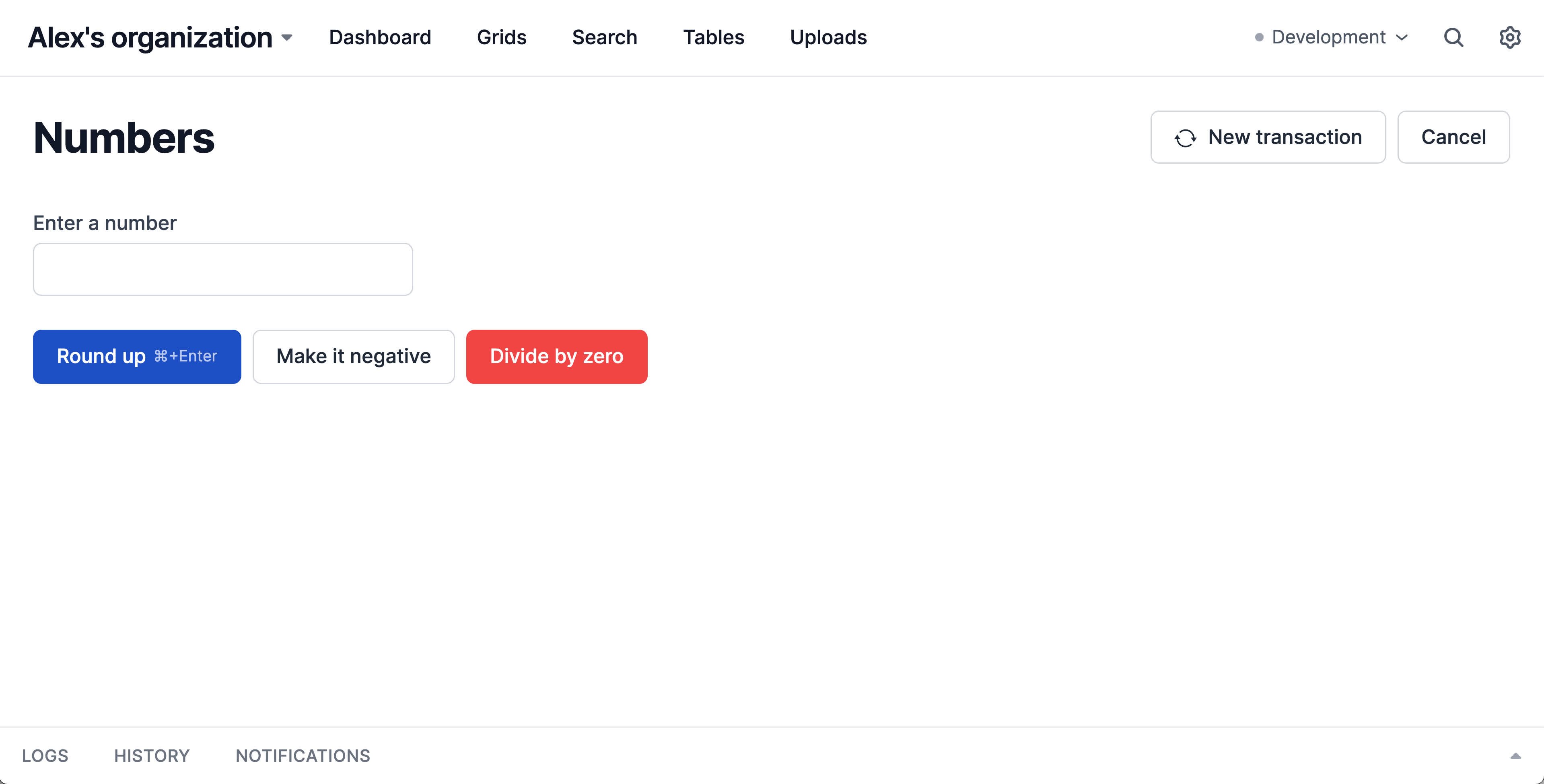
If you want the value returned as choice
to be different than the button label, or want to customize the button theme, you can customize it by passing an object with label
, value
, and optional theme
properties for the option.
The first option passed to withChoices
effectively serves as the default, and will be chosen if the person running the action submits the form with ⌘+Enter
or Ctrl+Enter
.
let { choice, returnValue } = await io.input
.number("Enter a number")
.withChoices([
"Round up",
{ label: "Make it negative", value: "negate", theme: "secondary" },
{ label: "Divide by zero", value: "divide-by-zero", theme: "danger" },
]);
if (choice === "Round up") {
returnValue = Math.ceil(returnValue);
}
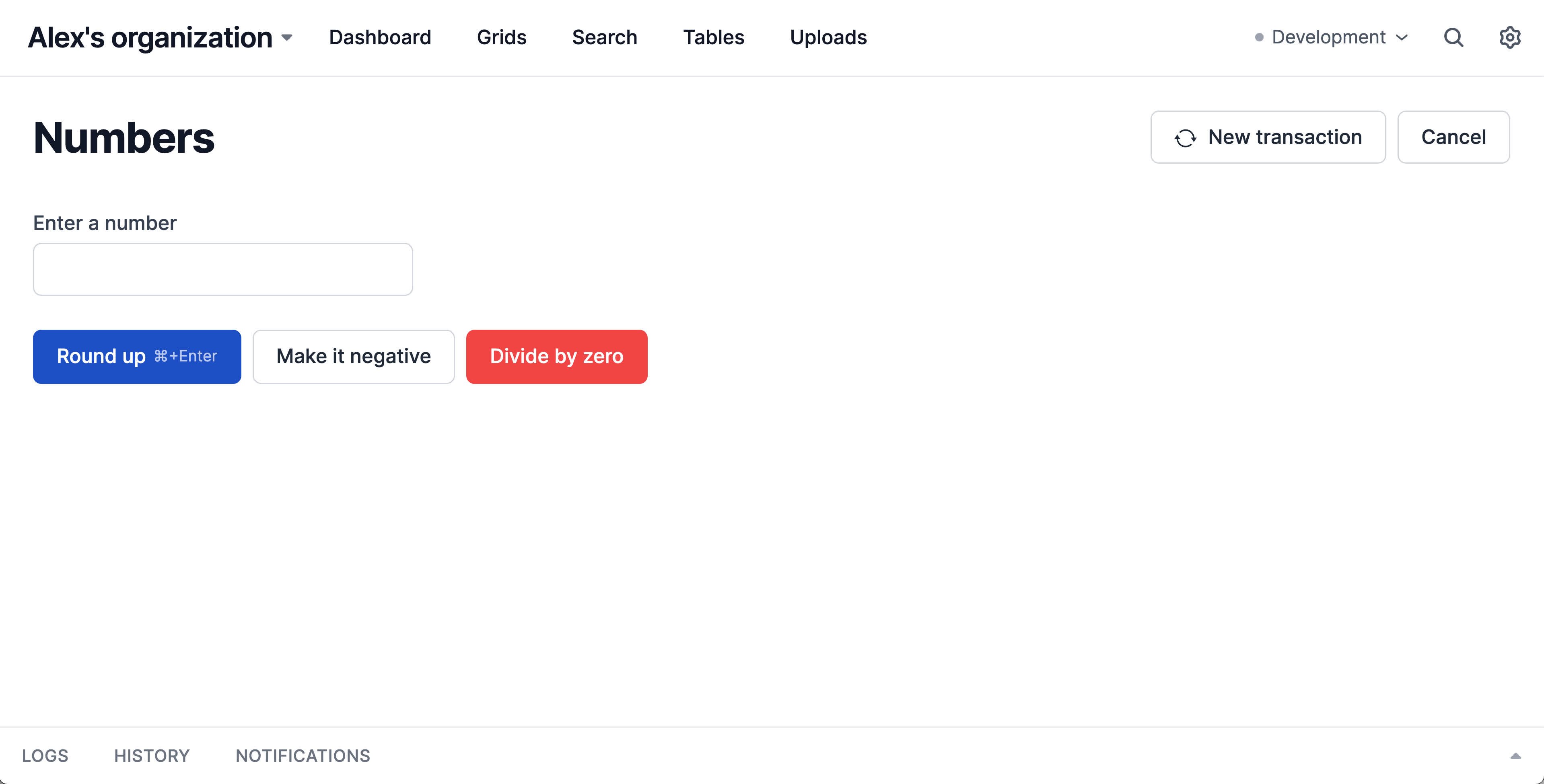
If you want the value returned as choice
to be different than the button label, or want to customize the button theme, you can customize it by passing an object with label
, value
, and optional theme
properties for the option.
The first option passed to withChoices
effectively serves as the default, and will be chosen if the person running the action submits the form with ⌘+Enter
or Ctrl+Enter
.
response = await io.input.number("Enter a number")
.with_choices([
"Round up",
{ "label": "Make it negative", "value": "negate", "theme": "secondary" },
{ "label": "Divide by zero", "value": "divide-by-zero", "theme": "danger" },
])
if response.choice == "Round up":
response.return_value = math.ceil(response.return_value)
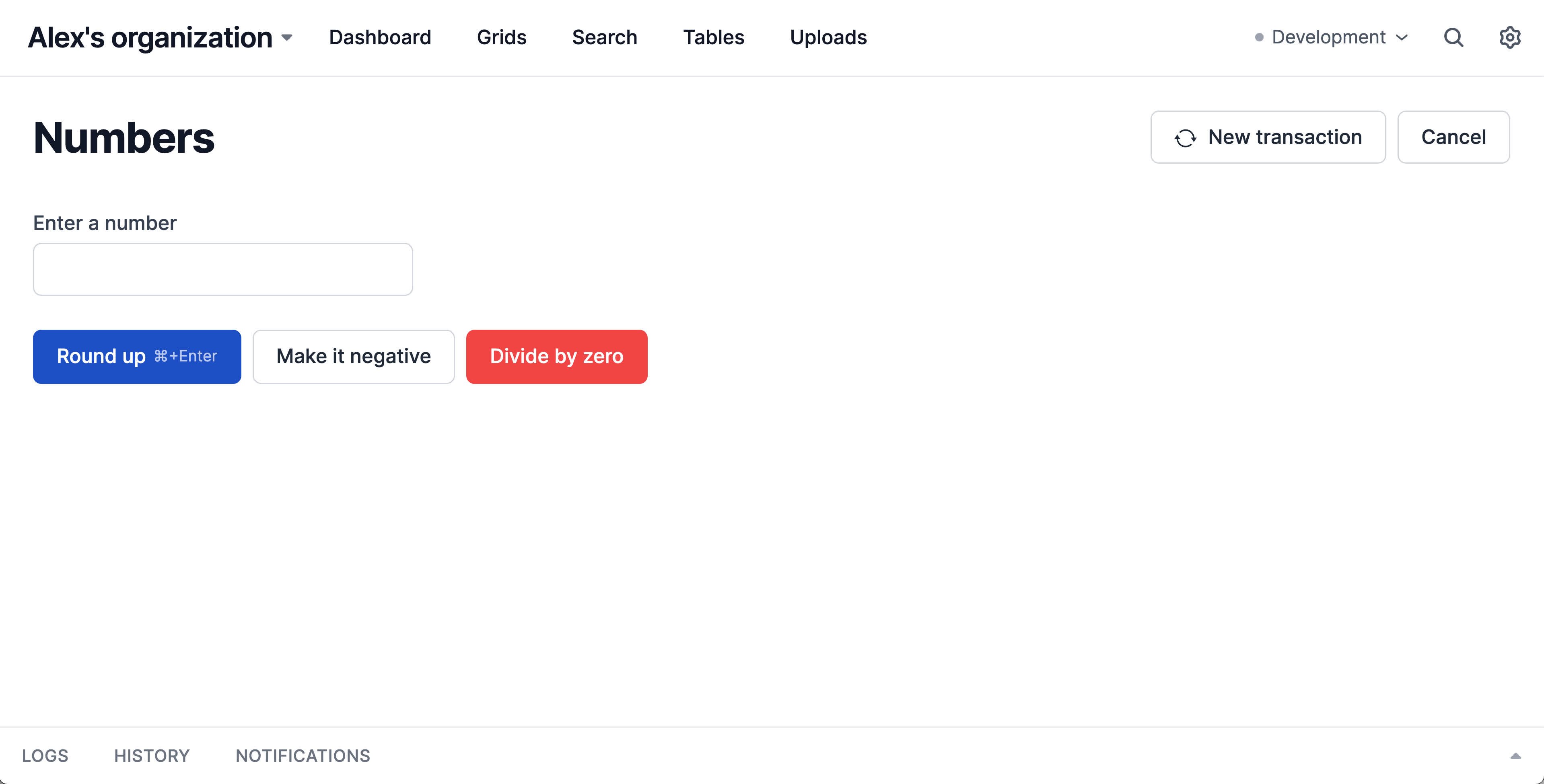
If you want the value returned as choice
to be different than the button label, or want to customize the button theme, you can customize it by passing an object with label
, value
, and optional theme
properties for the option.
The first option passed to with_choices
effectively serves as the default, and will be chosen if the person running the action submits the form with ⌘+Enter
or Ctrl+Enter
.
Usage with io.group
- TypeScript
- JavaScript
- Python
Like with other IO methods, the submit buttons for an io.group
can be customized with the
.withChoices()
chained method.
const {
choice,
returnValue: { num1, num2 },
} = await io
.group({
num1: io.input.number("First number"),
num2: io.input.number("Second number"),
})
.withChoices(["Add", "Subtract", "Multiply"]);
Like with other IO methods, the submit buttons for the group can be customized with the
.withChoices()
chained method.
const {
choice,
returnValue: { num1, num2 },
} = await io
.group({
num1: io.input.number("First number"),
num2: io.input.number("Second number"),
})
.withChoices(["Add", "Subtract", "Multiply"]);
Like with other IO methods, the submit buttons for the group can be customized with the
.with_choices()
chained method.
response = await io.group(
num1=io.input.number("First number"),
num2=o.input.number("Second number"),
).with_choices([
"Add",
"Subtract",
"Multiply",
]);